Points2D¶
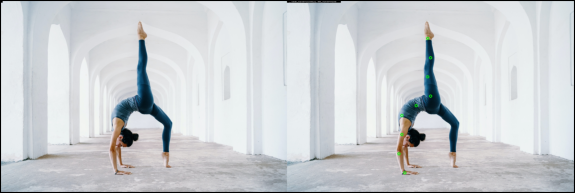
- class aloscene.points_2d.Points2D(x: Union[list, numpy.array, torch.Tensor], points_format: str, absolute: bool, labels: Optional[Union[dict, aloscene.labels.Labels]] = None, frame_size=None, names=('N', None), *args, **kwargs)¶
Bases:
aloscene.tensors.augmented_tensor.AugmentedTensor
Points2D Augmented Tensor. Used to represents 2D points in space encoded as xy or yx. The data must be at least 2 dimensional (N, None) where N is the number of points.
If your’re data is more than 2 dimensional you might need to set the names to (“B”, “N”, None) for batch dimension or (“T”, “N”, None) for the temporal dimension, or even (“B”, “T”, “N”, None) for batch and temporal dimension.
Warning
It is important to note that on Frame, Points2D are not mergeable by default . Indeed, one Frame is likely to get more or less points than an other one. Therefore, if you want to create Points2D with batch dimension, it is recommded to create Points2D tensors as part of a list that enclose the batch dimension (same for the temporal dimension).
>>> [Points2D(...), Points2D(...), Points2D(...)].
Finally, batch & temporal dimension could also be stored like this:
>>> [[Points2D(...), Points2D(...)], [Points2D(...), Points2D(...)]]
- Parameters
- x: list | torch.Tensor | np.array
Points2D data. See explanation above for details.
- points_format: str
One of “xy”, “yx”. Whether your points are stored as “xy” or “yx”.
- absolute: bool
Whether your points are encoded as absolute value or relative values (between 0 and 1). If absolute is True, the frane size must be given.
- frame_size: tuple
(Height & Width) of the relative frame.
- names: tuple
Names of the dimensions : (“N”, None) by default. See explanation above for more details.
Notes
Note on dimension:
C refers to the channel dimension
N refers to a dimension with a dynamic number of element.
H refers to the height of a SpatialAugmentedTensor
W refers to the width of a SpatialAugmentedTensor
B refers to the batch dimension
T refers to the temporal dimension
Examples
>>> pts2d = aloscene.Points2D( ... [[0.5, 0.5], [0.4, 0.49]], ... points_format="yx", absolute=False ....) >>> pts2d = aloscene.Points2D( ... [[512, 458], [28, 20]], ... points_format="yx", absolute=True, frame_size=(1200, 1200) )
- FORMATS = ['xy', 'yx']¶
- abs_pos(frame_size)¶
Get a new Point2d Tensor with absolute position relative to the given frame_size.
- Parameters
- frame_size: tuple
Frame size associated with the absolute points2d. (height, width)
Examples
>>> points_2d_abs = points.abs_pos((height, width))
- Return type
- append_labels(labels, name=None)¶
Attach a set of labels to the points. The attached set of labels are supposed to be equal to the number of points. In other words, the N dimensions must match in both tensor.
- Parameters
- labels: aloscene.Labels
Set of labels to attached to the frame
- name: str
If none, the label will be attached without name (if possible). Otherwise if no other unnamed labels are attached to the frame, the labels will be added to the set of labels.
Examples
>>> pts2d = aloscene.Points2D([[0.5, 0.5], [0.2, 0.1]], "yx", False) >>> labels = aloscene.Labels([51, 24]) >>> pts2d.append_labels(labels) >>> pts2d.labels >>> Or using named labels >>> pts2d = aloscene.Points2D([[0.5, 0.5], [0.2, 0.1]], "yx", False) >>> labels_set_1 = aloscene.Labels([51, 24]) >>> labels_set_2 = aloscene.Labels([51, 24]) >>> pts2d.append_labels(labels_set_1, "set1") >>> pts2d.append_labels(labels_set_2, "set2") >>> pts2d.labels["set1"] >>> pts2d.labels["set2"]
- fit_to_padded_size()¶
This method can be usefull when one use a padded Frame but only want to learn on the non-padded area. Thefore the target points will remain unpadded while keeping information about the real padded size.
Therefore. If the set of points did not get padded yet by the pad operation, this method wil pad the points to the real padded size.
Examples
>>> padded_points = points.fit_to_padded_size()
- Return type
- get_view(frame=None, size=None, labels_set=None, **kwargs)¶
Create a view of the points on a frame
- Parameters
- frame: aloscene.Frame
Tensor of type Frame to display the points on. If the frame is None, an empty frame will be create on the fly. If the frame is passed, the frame should be 3 dimensional (“C”, “H”, “W”) or (“H”, “W”, “C”)
- size: (tuple)
(height, width) Desired size of the view. None by default
- labels_set: str
If provided, the points will rely on this label set to display the points color. If labels_set is not provie while the points have multiple labels set, the points will be display with the same colors.
Examples
>>> points.get_view().render() >>> # Or using a frame to render the points >>> points.get_view(frame).render()
- get_with_format(points_format)¶
Get the points with the desired format.
- Parameters
- points_format: str
One of (“xy”, “yx”)
Examples
>>> n_points = points.get_with_format("yx")
- Return type
- rel_pos()¶
Get a new Point2d Tensor with relative position (between 0 and 1) based on the current frame_size.
Examples
>>> points_2d_rel = points.rel_pos()
- Return type
- remove_padding()¶
This method can be usefull when one use a padded Frame but only want to learn on the non-padded area. Thefore the target points will remain unpadded while keeping information about the real padded size.
Thus, this method will simply remove the memorized padded information.
- Returns:
[type]: [description]
- Return type
- xy()¶
Get a new Point2d Tensor with points following this format: [x, y]. Could be relative value (betwen 0 and 1) or absolute value based on the current Tensor representation.
Examples
>>> points_2d_xy = points.xy()
- Return type